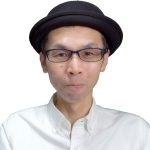
JavaScript の基本文法の配列についてまとめてみました。
配列(Array)
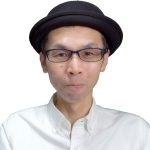
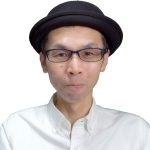
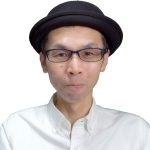
まずは基本文法から見ていきましょう。
const 配列の変数名 = [値1, 値2, 値3]
//配列リテラル:Arrayだと複数の値が入る
//各値には連番(index)が自動で振られる(0,1,2,3….)
console.log(配列名) //処理命令
Array(値の数)[値1, 値2, 値3] //返り値
console.log(配列名[index]) //処理命令
indexに該当する値 //返り値
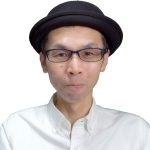
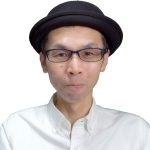
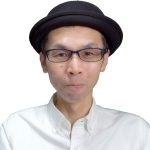
実際に使用例を見てみると…
値が数字の場合
const scores = [12, 35, 88] //配列リテラル:配列の変数名=scores
console.log(scores) //処理命令
Array(3)[ 12,35,88 ] //返り値
console.log(scores[0]) //処理命令:index0の値は?
12 //返り値
console.log(scores[2]) //処理命令:index2の値は?
88 //返り値
console.log(scores.length) //処理命令:格納されている値の数は?
3 //返り値
値が文字の場合
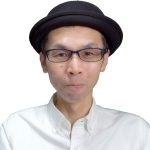
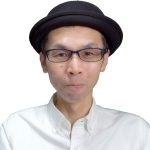
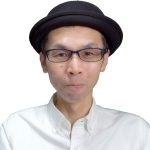
配列に文字を入れる場合は、” “を使います。
const letter = ["a", "松", "B"] //配列リテラル:配列の変数名=letter
console.log(letter) //処理命令
Array(3)[ "a","松","B" ] //返り値
console.log(letter[0]) //処理命令:index0の値は?
a //返り値
console.log(letter[1]) //処理命令:index1の値は?
松 //返り値
console.log(letter.length) //処理命令:格納されている値の数は?
3 //返り値
値の書き換え
配列でIndexを指定して値を変更すると配列が書き換わります。
配列名[index] = 書き換え後の値
const scores = [2, 4, 9, 11]
console.log(scores)
Array (4)[ 2,4,9,11 ]
scores[1] = 0 //値の書き換え:index1の値を0に
0
console.log(scores)
Array (4)[ 2,0,9,11 ]
値を足す場合
配列名.push(足す値)
配列名.push(数字)
配列名.push(“文字列”)
数字を足す場合は
const scores = [2, 4, 9, 11]
scores.push(90) //要素を足す:一番後ろに値90を足す
console.log(scores)
Array (5)[ 2,4,9,11,90 ]
文字列を足す場合は
const scores = [2, 4, 9, 11]
scores.push("山田") //値を足す:一番後ろに値 山田を足す
console.log(scores)
Array(5)[ 2,4,9,11,"山田" ]
真偽値(Boolean)を足す場合は
const scores = [2, 4, 9, 11]
scores.push(true) //値を足す:一番後ろに値 true(真偽値)を足す
console.log(scores)
Array(5)[ 2,4,9,11,true ]
配列に何個の値が入っているか?
配列名.length
const scores = [2, 4, 9, 11]
scores.length //配列scoresに入っている値数は?
4 //返り値
最後の要素を削除する方法
配列名.pop()
const scores = [2, 4, 9, 11]
scores.pop() //配列scoresの最後の値を削除
console.log(scores)
Array(3)[ 2,4,9 ]
最初の値を削除する方法
配列名.shift
const scores = [2, 4, 9, 11]
scores.shift() //配列scoresの最初の値を削除
console.log(scores)
Array(3)[ 4,9,11 ]
連想配列(Object)
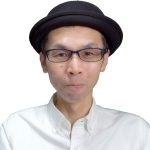
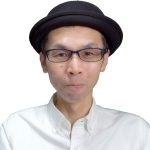
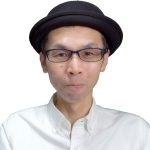
連想配列は複数のデータを管理できます。
const 連想配列の変数名 = {
キー: 値,
キー: 値,
キー: 値
}
例)
const scores = {
math: 70,
english: 80,
biology: 90
}
console.log(scores)
Object{ biology:90,english:80,math:70 }
配列から値の取り出す方法
連想配列の変数名[‘キー’]
const scores = {
math: 70,
english: 80,
biology: 90
}
scores['math'] //連想配列scoresからキーmathの値を取り出す
70 //返り値
配列の値を書き換える
連想配列の変数名.キー = 新しい値
const scores = {
math: 70,
english: 80,
biology: 90
}
scores.math = 100 //連想配列のキーmathの値を100に変える
console.log(scores)
Object{ biology:90,english:80,math:100 }
配列の中に連想配列を入れる
const 配列の変数名 = [
{ キー1: 値1, キー2: 値2, キー3: 値3 },
{ キー4: 値4, キー5: 値5, キー6: 値6 }
]
配列の変数名 [0] //index0の連想配列は?
Object { キー1: 値1, キー2: 値2, キー3: 値3 } //返り値
配列の変数名 [1] //index1の連想配列は?
Object { キー4: 値4, キー5: 値5, キー6: 値6 } //返り値
連想配列名[1].キー1 //index1の連想配列のキー4の値は
値4 //返り値
const scoresArray = [
{ math: 70, english: 80, biology: 90 },
{ math: 90, english: 70, biology: 80 }
]
scoresArray [0] //配列scoresArrayのindex0の値は?
Object { biology:90,english:80,math:70 }
scoresArray [1] //配列scoresArrayのindex1の値は?
Object { biology:80,english:70,math:90 }
scoresArray [1].english //配列scoresArrayのindex1のキーenglishの値は?
70
連想配列の中に関数を入れる
const user = {
lastName: '山田',
firstName: '花子',
fullName: function(){ //キーfullName:関数で定義されている
return`${this.lastName} ${this.firstName}` //this.キーでこの連想配列のキーの各値にアクセスできる
}
}
user.fullName() //連想配列userの関数で定義されているキーfullName()
山田 花子 //返り値
*参考1)関数式で定義された関数
*参考2)引数を必要としない関数
例2)関数を2つ含む場合
const user = {
lastName: '山田', //キー:値
firstName: '花子', //キー:値
fullName: function(){ //キー:関数
return`${this.lastName} ${this.firstName}` //関数の処理
},
sayHello: function() { //キー:関数
const fullName2 = this.fullName() //関数の処理 this.キーでこの連想配列のキーの各値にアクセスできる
console.log(`こんにちは${fullName2}です`) //関数の処理
}
}
user.sayHello() //連想配列userの関数で定義されているキーsayHello()
こんにちは山田 花子です
*注意)this.の使い方
繰り返し文|for文とforEach文
for文
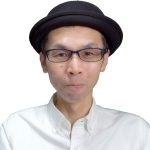
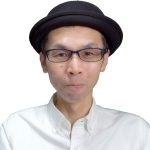
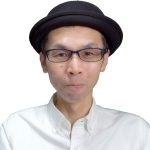
繰り返し処理を行うfor文の使い方から見ていきましょう。
for (変数 of 配列名) {
実行する処理;
}
const scores = [1,2,3,40,56,7,89,10,0] //配列の変数宣言: scores
for (let score of scores) { //配列をindexごとに一つ一つ分解する変数scoreの宣言
console.log(score) //変数scoreの順番に全て書き出し
}
//以下、返り値
1
2
3
40
56
7
89
10
0
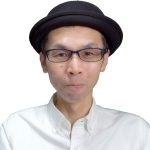
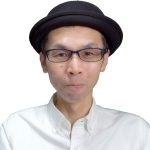
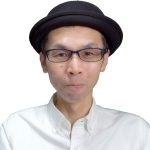
以下の例は実戦ではあまり出て来ませんが、たまに目にするので載せておきます。
const scores = [1,2,3,40,56,7,89,10,0] //配列の変数宣言: scores
for (let count = 0; count < 5; count++){ //最初count = 0が実行され、{}内の処理が実行されたら1をプラスし、5未満の値まで繰り返す
console.log(count)
}
//以下、返り値
0
1
2
3
4
forEach文
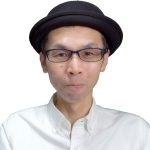
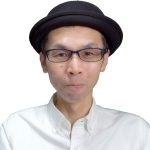
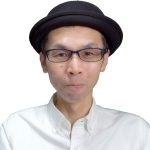
forEach文でもfor文と同じことができます。
配列名.forEach (function(変数) {
実行する処理;
})
const scores = [1,2,3,40,56,7,89,10,0] //配列の変数宣言: scores
scores.forEach(function(score){ //配列をindexごとに一つ一つ分解する変数scoreの定義
console.log(score)
})
//以下、返り値
1
2
3
40
56
7
89
10
0